In this article I am going to outline some actionable steps that can show you how to make a web page load faster. You can consider that to be some sort of on-page SEO. You probably know why that’s so important so I’m not going to get into that just to fill in a word quota.
In general you should load resources when they are actually needed. Nothing new, but there’s a lot of creativity to it because every web app is a different case.
The Google Maps case
If you ever used Google Maps on a web page and opened the DevTools, you probably saw that it loads nearly 1 MB of resources - scripts, small icons and fonts. So the way to solve this is by executing that Google Maps script when the user must see the map.
The first scenario is that if the map is located somewhere down the page - which means that the trigger for executing the script should be a certain scroll position. There’s a nice way to achieve that with Intersection Observer API:
function registerGoogleMaps(url) {
let target = document.getElementById('map');
let observer = new IntersectionObserver(function (entries) {
entries.forEach(function(entry){
if(entry.isIntersecting) {
let scriptEl = document.createElement('script');
scriptEl.setAttribute('src', url);
target.after(scriptEl);
observer.disconnect();
}
});
});
observer.observe(target);
};
The second scenario is if the map shows up when a button is clicked - the trigger here is obvious. Just execute that script when the click happens or some element gets into the page.
The third scenario is the trickiest and that is if the map is one of the first elements that the user sees (aka above the fold). Depending on the specific case, I think there are two ways of dealing with this:
- Use a map image instead of the real map and wait for some interaction - click, hover etc;
- Greatly defer the execution of the map script by showing some kind of loading (not nice)
The on-site chat case
On-site chat apps are not useless, that’s for sure. But they can also hinder your page load speed.
Essentially what you can do here is simulate the chat app until someone decides to interact with it.
The first scenario, for example, take this mobile version of the JivoChat instance: at the bottom right corner is the button that opens the chat. Now this button is not much - we can easily duplicate it with some html and css and position it where it needs to be.
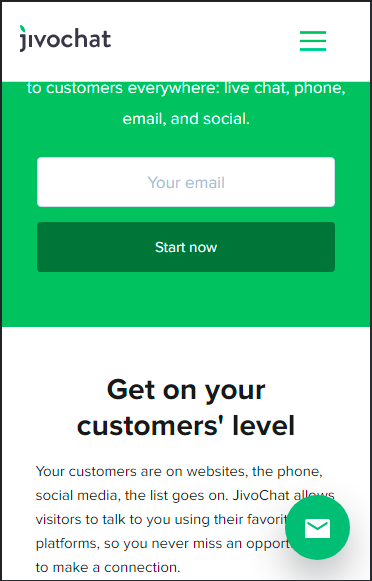
The same goes for the desktop version which displays the chat button differently.
After that we can initialize the chat when the user clicks it.
This has some potential drawbacks and it mostly relies on the fact that once you’ve set up your chat it won’t change often.
The second scenario is trickier - when you are using a proactive prompt - that’s when the chat pops up when you enter the website. It can be irritating, I know, but it’s effective.
Same principle applies here, however I’m not entirely sure how much you’ll gain compared to the amount of effort required and possible issues in the future. I haven’t really tried that and I don’t really recommend doing it.
If you are looking for an easier way to defer the chat loading you can just wait for any interaction from the user with your site. My idea here is that you can load the chat when the user scrolls for the first time or clicks somewhere, etc. You can even just postpone that script with a timeout for like 10 seconds because it’s not a critical resource to have on your website.
The images’ case
If you are the type of person that could benefit from the information here so far, you probably know most of the image optimization techniques out there for getting your page to load faster. If you don’t there are literally tons of resources, probably one of the best being web.dev - https://web.dev/fast/#optimize-your-images
All in all - load only compressed versions of those images that currently should be shown and try to figure out if the whole image is needed or just a part of it.
That last bit is hard to achieve unless your website is a small blog like this one and you are able to process every image by hand. There are a number of services that can help with automating the optimization process, one of them being Cloudflare’s - https://developers.cloudflare.com/images/cloudflare-images/
However, that’s a paid solution and may not be what you’re looking for.
The CSS frameworks case
I have to admit - I’m not really up to date with the latest CSS frameworks like Tailwind, but I know the good old Bootstrap and what it brings to the table.
That’s not going to be a rant to learn CSS Grid or why you don’t need Bootstrap. I use it almost everywhere but I often find myself using the grid system and some utility classes. Probably 90% of the CSS I bring with Bootstrap is unused. Now I know that if it were javascript, there are treeshaking techniques that can remove unused code, but for CSS I’m not currently aware of such.
So, when using Bootstrap, ask yourself “What do I actually use from this framework?” and try to isolate those modules in order to trim down the CSS size.
Same goes for any other framework.
The fonts case
For a thorough guide on optimizing fonts, again - web.dev https://web.dev/fast/#optimize-webfonts
Although browsers have nice fonts built-in, if you really need to load your custom font, please make sure you’re not using that just for a single word, like for typing out your logo or just a paragraph. Furthermore, decide if you really need that extra font weight besides the normal and bold ones.